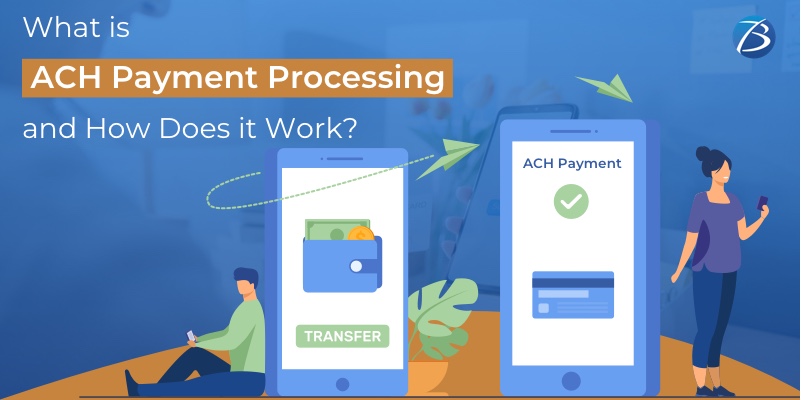
What is ACH Payment Processing?
What are the different types of ACH transactions?
- Direct payments & IRS payments
- eCheque transactions
- One-time or recurring payments into an IRA (Individual Retirement Account), savings account, taxable brokerage account, etc.
- Funds transfer between banks using payment gateways like PayPal, Venmo, etc.
- Businesses paying vendors and suppliers for products/services
- Businesses receiving payments from customers/clients
- Online bill payment through bank accounts
- Employees receiving direct salary deposits from employers
How do ACH Transfers differ from Wire Transfers?
How to implement the ACH Payment Model via the ACH Network?
How to integrate ACH Payment Processing?
# Importing the essential libraries
import requests
import json
# Configuringthe endpoint URL
ENDPOINT_URL = https://api.example.com/ach/payments
#Setting up the API key
API_KEY = “your_api_key_here”
# Configuring the bank account information of the sender and the recipient
sender_account = {
“account_number”: “1234567890”,
“routing_number”: “123456789”,
“account_type”: “checking”
}
recipient_account = {
“account_number”: “0987654321”,
“routing_number”: “987654321”,
“account_type”: “savings”
}
# Configuring the amount to be paid
amount = 100.00
# Generating the payload for executing the API request
payload = {
“sender_account”: sender_account,
“recipient_account”: recipient_account,
“amount”: amount
}
# Configuring the API request headers
headers = {
“Content-Type”: “application/json”,
“Authorization”: f”Bearer {API_KEY}”
}
# Sending the API request for payment processing
response = requests.post(ENDPOINT_URL, json=payload, headers=headers)
# Verifying the status code of the response
if response.status_code == 200:
# Generating the transaction ID if the payment processing gets successfully executed
transaction_id = response.json()[“transaction_id”]
print(f”Payment processed successfully. Transaction ID: {transaction_id}”)
else:
# Generating the error message, if the payment processing fails
error_message = response.json()[“error”]
print(f”Payment failed. Error: {error_message}”)
ACH Payment: Benefits for Businesses & Consumers
Cost-Savings
Speedy, Hassle-free, & Accurate Payment Processing
Automates Recurring Transactions
High-end Security
Hassle-free Payment Transaction Management
Are You Interested in Building a Top-Class Website or Mobile App?