Significant Data Storage Mechanisms in React Native Applications
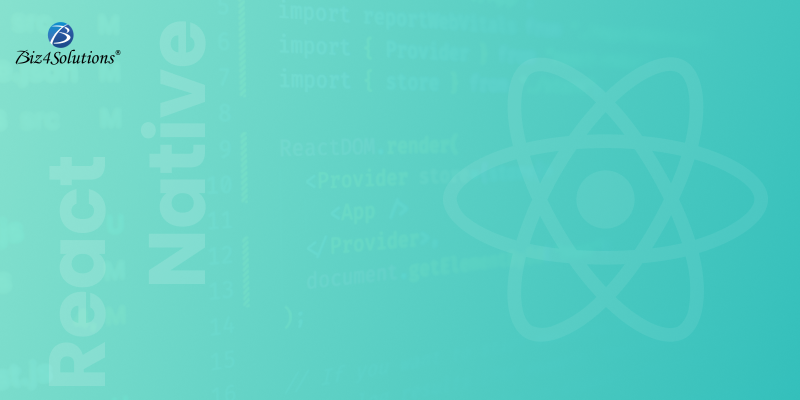

Storage Options for React Native App Data

Async Storage
MMKV Storage
- MMKV is compatible with both iOS and Android platforms, making it ideal for cross-platform React Native applications. It provides direct bindings to the native C++ library, accessible through a straightforward JavaScript API.
- Engineered for speed, MMKV boasts quicker write/read speeds compared to SQLite and Async Storage. Despite its impressive performance, MMKV maintains a small footprint and demands minimal overhead, making it an excellent choice for resource-constrained environments.
- React Native applications often require a storage mechanism that persists data across application restarts or device reboots. MMKV supports various types of data and offers encryption for secure storage. Additionally, it supports multiple instances, allowing for the separation of user-specific and global data.
- MMKV prioritizes stability and reliability, aiming to prevent data loss and corruption. Its library facilitates easy storage and retrieval of data using a simple API, supporting maps, arrays, and strings. Memory synchronization with a file is achieved through mmap, and value encoding or decoding is performed using protobuf.
- MMKV is user-friendly, eliminating the need for complex configurations. It automatically saves modifications without requiring explicit synchronized calls.
npm install react-native-mmkv-storage
import MMKVStorage from 'react-native-mmkv-storage';
const storage = new MMKVStorage.Loader().initialize(); // Store data
await storage.set(‘myKey’, ‘myValue’); // Retrieve data
const value = await storage.get(‘myKey’); SQLite
npm install --save react-native-sqlite-storage
yarn add react-native-sqlite-storage
import SQLite from 'react-native-sqlite-storage';
// Open a database
const databaseConfig = {
name: ‘mydb.db’,
location: ‘default’
}; const db = SQLite.openDatabase(databaseConfig);
// Create a table
db.transaction((tx) => {
tx.executeSql(
CREATE TABLE IF NOT EXISTS items (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT,
quantity INTEGER
);
[],
() => {
console.log(‘Table created successfully’);
},
(error) => {
console.log(‘Error creating table: ‘ + error.message);
},
);
}); // Insert data into the table
db.transaction((tx) => {
tx.executeSql(
‘INSERT INTO items (name, quantity) VALUES (?, ?)’,
[‘Apples’, 10],
() => {
console.log(‘Data inserted successfully’);
},
(error) => {
console.log(‘Error inserting data: ‘ + error.message);
},
);
}); Realm
import Realm from 'realm';
const realm = new Realm({
schema: [{ name: ‘Person’, properties: { name: ‘string’ } }],
}); realm.write(() => {
realm.create(‘Person’, { name: ‘John’ });
}); const people = realm.objects(‘Person’); console.log(people); Firebase Realtime Database
npm install firebase
// firebase.js
import firebase from 'firebase';
const firebaseConfig = {
// Your Firebase project config goes here
}; firebase.initializeApp(firebaseConfig); export default firebase; // Any component or file where you need to write data
import firebase from './firebase';
// Write data to the database
firebase.database().ref(‘users/1’).set({
name: ‘John Doe’,
email: ‘johndoe@example.com’,
}); // Any component or file where you need to read data
import firebase from './firebase';
// Listen for changes to a particular data path
firebase.database().ref(‘users/1’).on(‘value’, (snapshot) => {
const user = snapshot.val();
console.log(user);
}); Are You Interested in Building a Top-Class Website or Mobile App?