
Key Steps to test animated views in iOS
Identify the Animated View and its Components
- Identify the type of animation you intend to use in the app -zoom in/zoom out, fade in/fade out, slide in/slide out, etc.
- Set the duration time of the animation – the time taken for the completion of the animation. Decide on this time very carefully as it determines the effect of your animation.
- Identify the specific user interactions or the particular events that will trigger the animation to function. Examples of such triggers include scrolling, button tapping, etc.
- Examine how accurately and precisely the animation will be working or behaving.
- Check how the animation is impacting the app’s CPU, performance, and memory usage.
- Consider the feedback app users will get during and after the animation – visual indicators or haptic feedback.
Set up the Test Environment
Write Unit Tests for the underlying logic of the iOS App
Debug using the in-built Debug Tools for Animations in Xcode
What are the different Methodologies to test Animated Views in iOS?
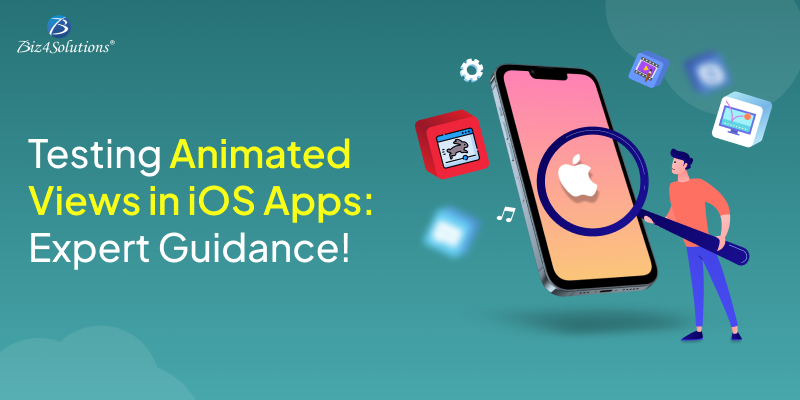
Asynchronous Testing
let animationExpectation = XCTestExpectation(description: “Animation completed”)
// Start animation code here
// After the animation is completed, fulfill the expectation
animationCompletionHandler = {
animationExpectation.fulfill()
}
// Wait till the expectation gets fulfilled
wait(for: [animationExpectation], timeout: 5)
// Start animation code here
// At the end of the animation, perform the test
animationCompletionHandler = {
// Perform test here
}
// Start animation code here
// At the end of the animation, perform the test on the main queue
animationCompletionHandler = {
DispatchQueue.main.async {
// Perform test here
}
}
Manual Testing
Automated Testing
func testButtonAnimation() {
let app = XCUIApplication()
app.launch()
let button = app.buttons[“myButton”]
button.tap()
// Verify the animation
let animationExpectation = expectation(description: “Button animation completed”)
DispatchQueue.main.asyncAfter(deadline: .now() + 1.0) {
XCTAssertTrue(button.frame.origin.x > 0, “Button should move to the right during animation”)
animationExpectation.fulfill()
}
waitForExpectations(timeout: 2.0, handler: nil)
}
Are You Interested in Building a Top-Class Website or Mobile App?