Welcome to the world of Angular, a robust framework designed to build dynamic, high-performance web applications. Angular is a platform that empowers developers to create seamless user experiences by leveraging a range of tools and services within its ecosystem. From its powerful CLI to its modular structure, Angular provides a comprehensive environment for professional-grade application development.
Understanding how an Angular application works is key to harnessing its full potential. This framework, developed by Google, is known for its ability to assist developers in managing both the construction and the scaling of single-page applications. Angular achieves this through its use of TypeScript—a superset of JavaScript, which ensures higher security, easier maintainability, and better development workflows.
Angular’s ecosystem includes a variety of integrated libraries, real-time testing capabilities, and a dedicated community that contributes to its ever-evolving nature. This article aims to demystify the structure, components, and lifecycle of an Angular application, providing you with a clear blueprint of its internal workings.
Core Components of an Angular Application
At the heart of any Angular application lies a set of core components that act as the building blocks for its architecture. These components are integral to understanding how an Angular application works. An Angular application is essentially a tree of components, starting with a root component that branches out into various feature components to form a hierarchical structure.
The primary components include:
- Modules: They organize the application into cohesive blocks of functionality. Each Angular app has at least one module, the root module, which is conventionally named AppModule.
- Components: They are the basic UI building blocks of the Angular app. Each component consists of a template, which defines the view, and a class that manages the view’s logic.
- Services: Reusable data services are often injected into components to share code and functionality across the application.
- Templates: Written with HTML, templates form the view for Angular’s components and can include Angular-specific template syntax to enhance functionality.
- Metadata: Decorators are used to add metadata to classes so that Angular understands how to process them.
- Data Binding: Angular’s data binding features allow for the seamless synchronization between the model and the view components.
- Directives: These are classes that add additional behavior to elements in your Angular applications.
- Dependency Injection: Angular’s powerful DI framework provides components with the services they require, enhancing modularity and reusability.
Understanding the role and function of each component within this ecosystem is crucial for developers to effectively build and maintain scalable web applications. The synergy between these components results in a reactive system where each part works in concert with the others, providing a smooth development experience and a high-performance end product.
Understanding the Angular Module System
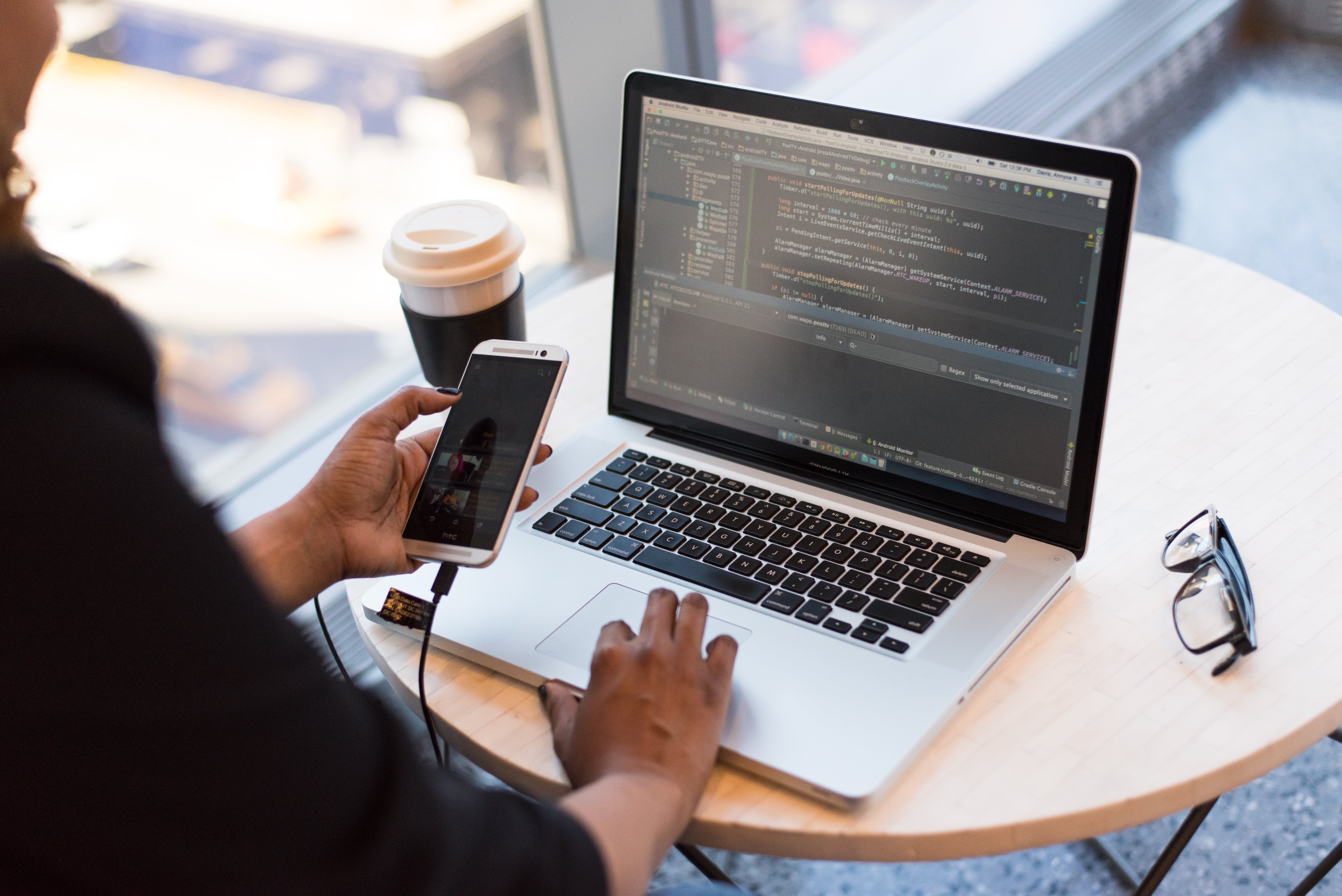
The module system is a fundamental concept that dictates how an Angular application works. An Angular module, known as an NgModule, is a way to consolidate related code into functional sets; it’s like a container for a cohesive group of code dedicated to an application domain, a workflow, or a closely related set of capabilities.
An Angular application may have several modules, each with a specific responsibility. The root module, typically referred to as AppModule, is where you bootstrap the application to launch it, while feature modules are focused on specific application features, like user authentication or data management.
Modules serve several key purposes:
- They help in organizing the application into chunks that can be developed and tested independently.
- They can be lazy-loaded to improve performance, which means that Angular only loads them as they are needed, rather than at the start.
- They promote code reusability by grouping functionalities that can be imported into other modules.
Each Angular module can contain:
- Components: Which define views, pieces of screen that Angular can choose and modify according to your program logic and data.
- Services: Which provide specific functionality not directly related to views and are intended to be shared across components.
- Directives, Pipes, and Providers: That can change the behavior of components, transform displayed values, and inject dependencies respectively.
By properly understanding and utilizing the Angular module system, developers are empowered to create highly organized, maintainable, and efficient applications. The module system is critical in managing the scope of the application’s components, services, and directives, and facilitates the division of work across different team members and scalability of the application over time.
The Angular Component Lifecycle Explained
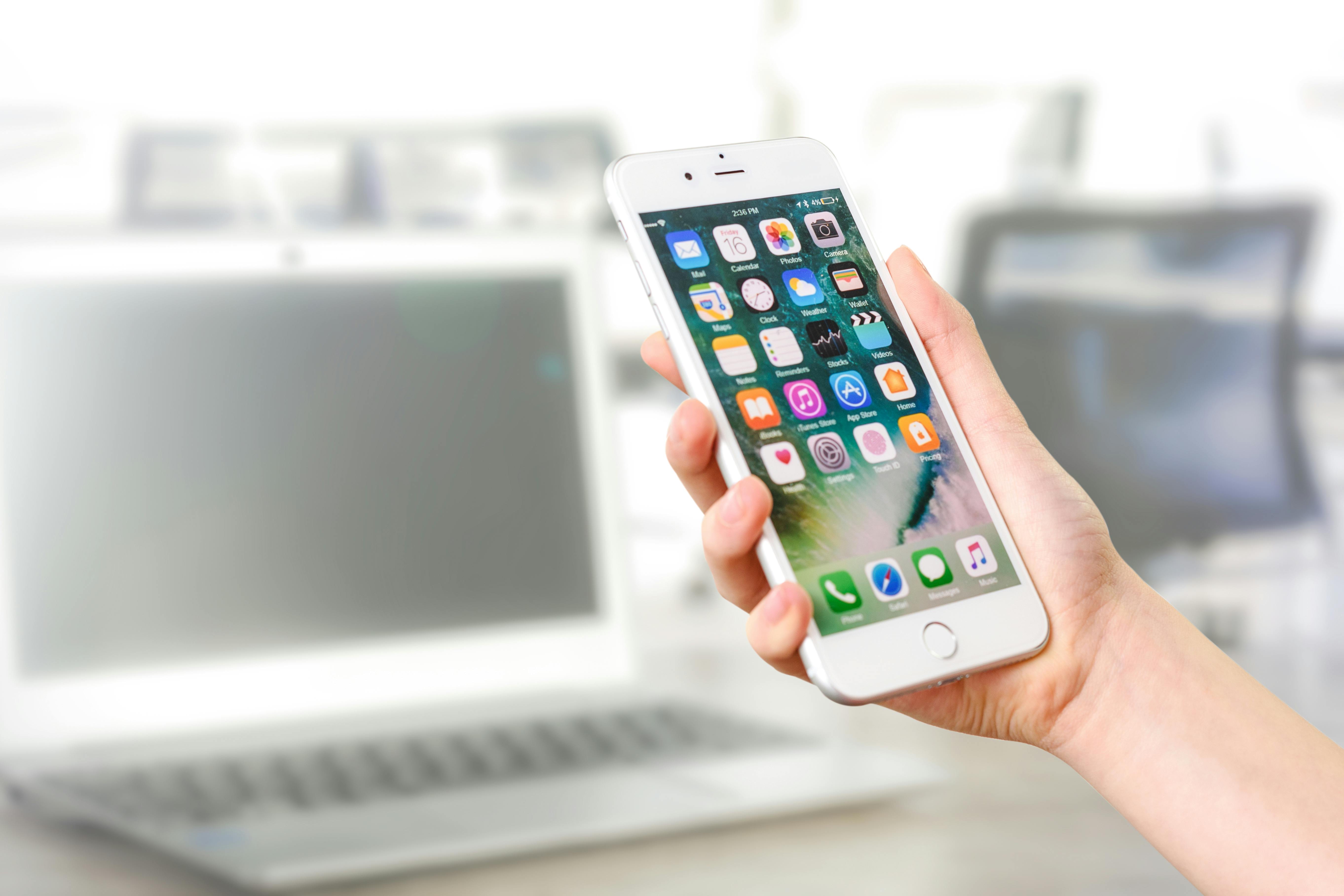
In understanding how an Angular application works, it is crucial to grasp the concept of the component lifecycle. Each Angular component goes through a series of lifecycle events from creation to destruction, which you can tap into to influence the behavior of your application at different points in time.
The Angular framework provides lifecycle hooks that give visibility into these key moments and allows you to perform custom logic. Some of the primary lifecycle hooks include:
ngOnInit
: Executed after the firstngOnChanges
, it is used for initializing the component.ngOnChanges
: Called whenever there is a change in one of the input properties of the component.ngDoCheck
: A hook for manual change detection when Angular’s automatic change detection doesn’t cover your needs.ngAfterContentInit
: Responds after Angular projects external content into the component’s view.ngAfterContentChecked
: Responds after Angular checks the content projected into the component.ngAfterViewInit
: Responds after Angular initializes the component’s views and child views.ngAfterViewChecked
: Responds after Angular checks the component’s views and child views.ngOnDestroy
: Called just before Angular destroys the component, useful for cleanup.
These hooks offer developers the flexibility to add their own code at specific points in the lifecycle of a component. For example, you might want to load data from an API during ngOnInit
as the component is being initialized, or you may need to unsubscribe from observables when the component is destroyed to prevent memory leaks using ngOnDestroy
.
Proper utilization of these lifecycle hooks is essential for creating dynamic and responsive applications. They allow developers to optimize performance and ensure that the application responds appropriately as users interact with it and as data changes over time.
Dependency Injection in Angular Applications
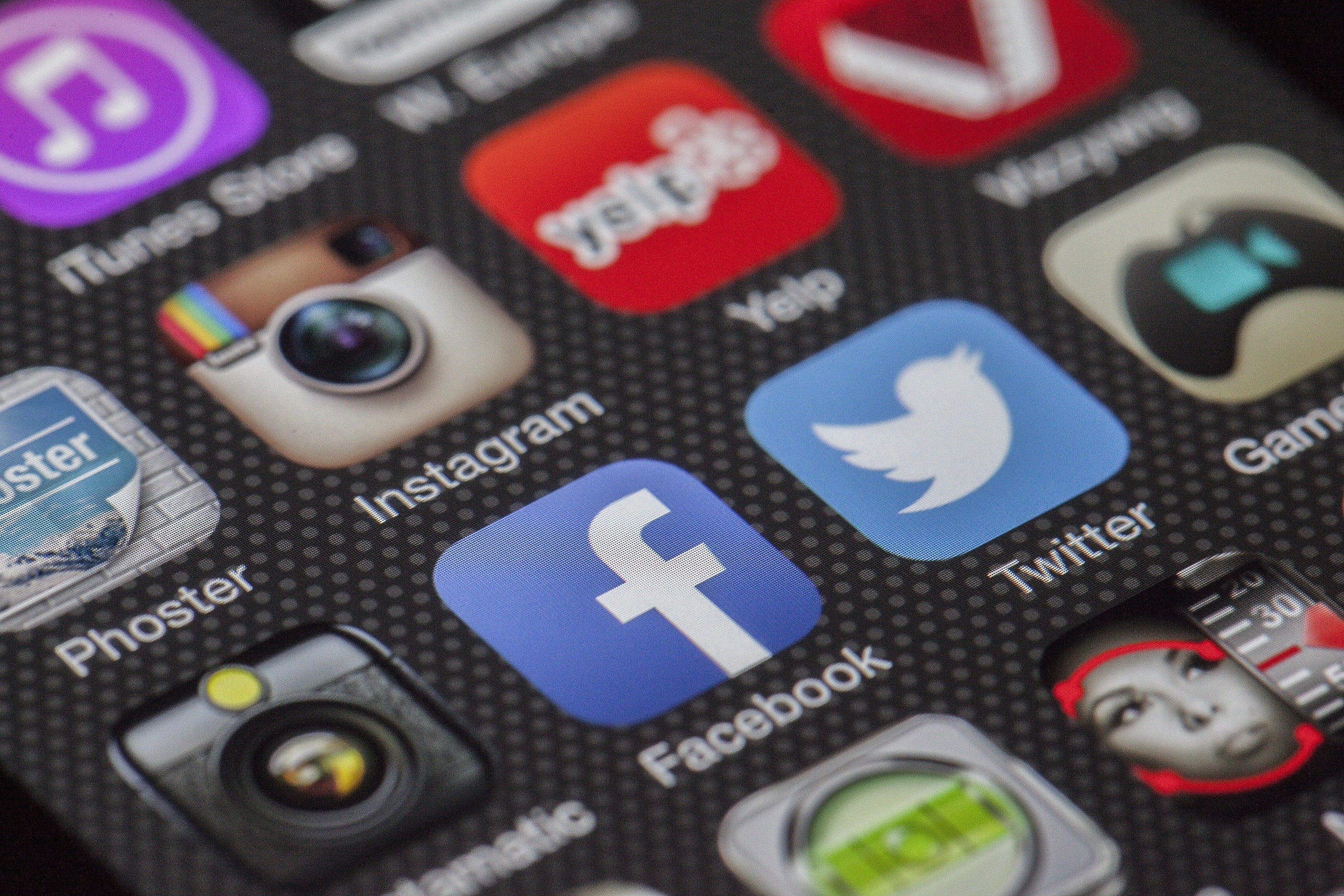
One of the most powerful features in Angular is its dependency injection (DI) system. Dependency Injection in Angular applications decouples the way in which objects obtain their dependencies. In Angular, DI is a design pattern in which a class requests dependencies from external sources rather than creating them itself.
At its core, Angular’s DI framework provides a robust and efficient way to manage the creation and delivery of services. The injector is the main hub of the DI system and is responsible for creating service instances and injecting them into classes like components and services.
To utilize DI, services are typically decorated with the @Injectable()
decorator, signaling to Angular that they can be injected into other classes. When a component requires a particular service, it is added to the component’s constructor in the form of a parameter:
constructor(private myService: MyService) { }
When Angular creates a component, it will look at the constructor parameters and provide the requested services if available in the application’s injector. This system has several benefits:
- Reduces class coupling and increases modularity.
- Makes unit testing easier by allowing you to provide mock services.
- Improves application maintainability and scalability.
DI is not limited to services; it can also be used to inject values and functions into classes. Custom providers can be created to further control how and where services are instantiated, allowing for a more flexible and scalable application architecture. The DI system in Angular is a testament to the framework’s commitment to creating maintainable and efficient applications.
Routing and Navigation in Angular
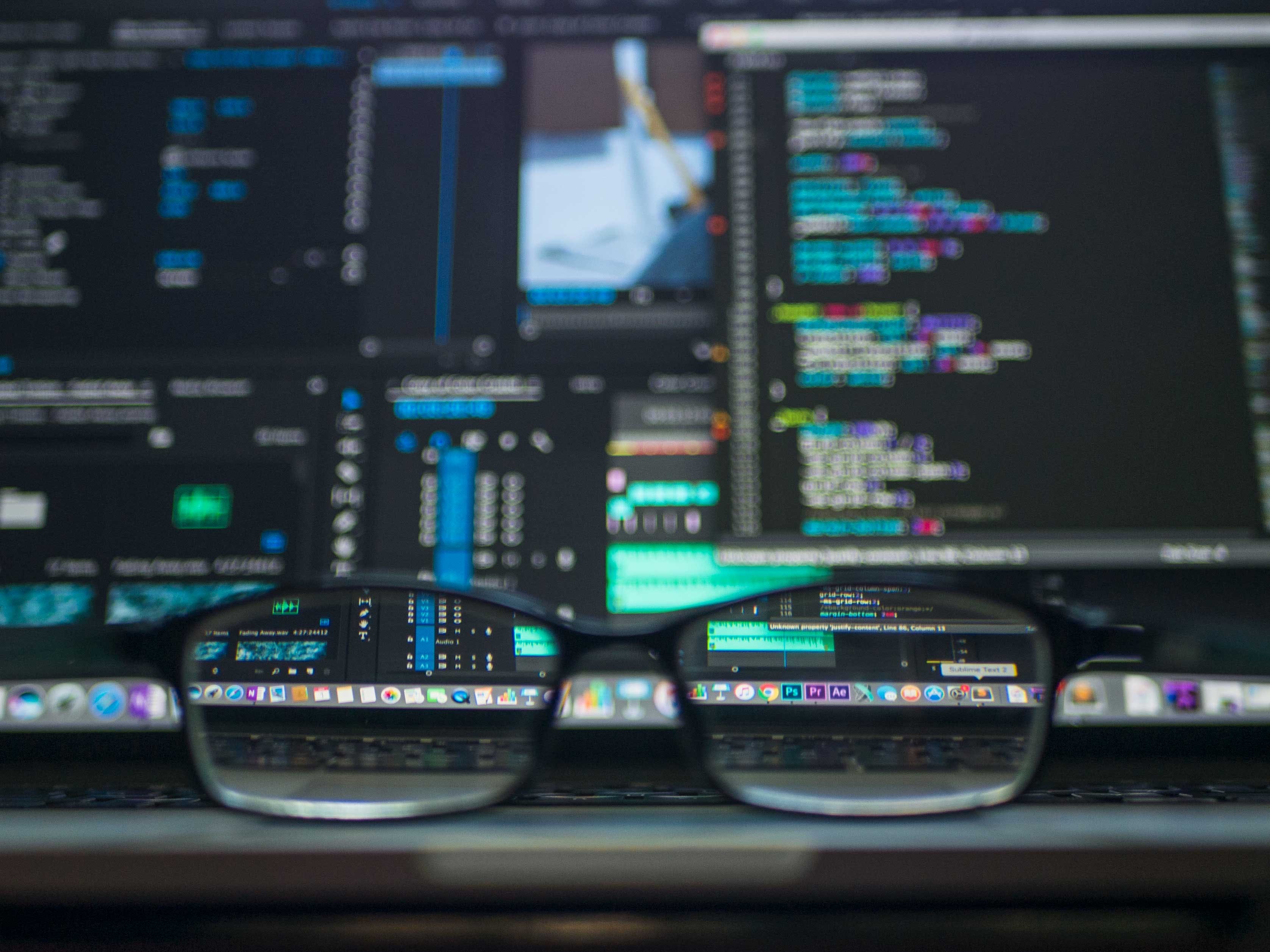
Angular’s routing and navigation capabilities provide a way to define paths that correspond to different components in an application. Through the RouterModule
, Angular allows developers to set up navigation paths for a seamless user experience. A router outlet within the application’s template acts as a placeholder that Angular dynamically fills based on the current navigation state.
Each route in Angular can have both a path and a component associated with it, defined in an array of routes. This array is then passed to RouterModule.forRoot()
in the root module, or RouterModule.forChild()
for feature modules, which configures the router to use the specified routes:
const appRoutes: Routes = [
{ path: 'feature', component: FeatureComponent },
// other routes
];
Are You Interested in Building a Top-Class Website or Mobile App?
Navigation is performed using the Router
service, which provides methods like navigate()
and navigateByUrl()
, allowing applications to switch between views programmatically. Angular’s routing also supports route parameters, query parameters, and wildcards, offering a high level of customization for handling various navigation scenarios.
Angular’s approach to routing ensures that applications are SEO-friendly, as each view can have its own URL, which is crucial for indexability. With lazy loading, developers can also improve performance by loading feature modules on demand, rather than at the initial loading of the application.
Routing is essential for creating single-page applications (SPAs) that need to handle complex navigation without the need for full page refreshes. It’s a cornerstone of modern web applications, and Angular provides a sophisticated, yet straightforward, framework for managing navigation.